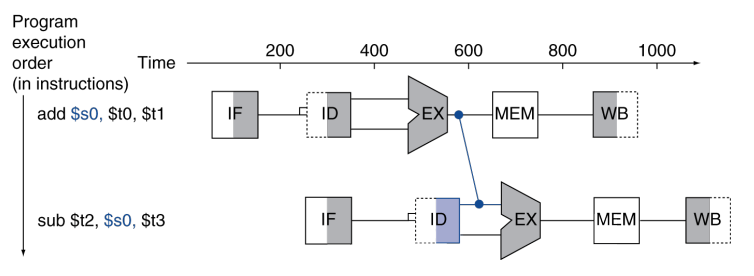
## Forwarding (aka Bypassing)
- Forwarding cannot always resolve a data hazard
```mips
lw $s0, 20($t1)
sub $t2, $s0, $t3
```
- What stage does `lw` produce the bits of `$s0`?
+
After Stage 4: MEM
## Forwarding (aka Bypassing)
- Why does this create an unavoidable stall?
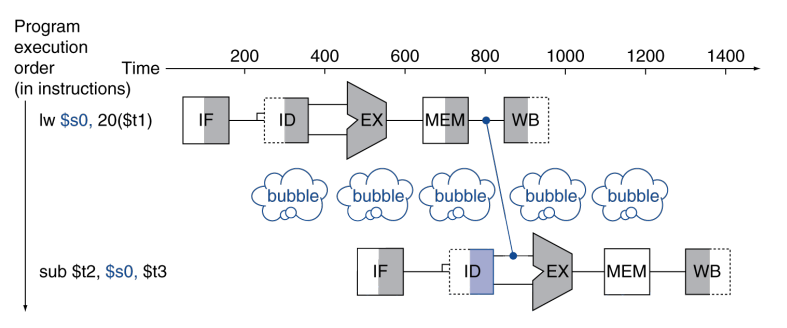
-
We cannot send data **backwards in time**
## Data Hazard Exercise
- Consider the following MIPS code:
```mips
lw $t0, 40($a3)
add $t6, $t0, $t2
sw $t6, 40($a3)
```
-
Assuming there is no forwarding implemented, are any stalls necessary?
-
How many clock cycles are required to execute these three lines of code without forwarding?
## Data Hazard Exercise
- Consider the following MIPS code:
```mips
lw $t0, 40($a3)
add $t6, $t0, $t2
sw $t6, 40($a3)
```
- Assuming there IS forwarding implemented, are any stalls necessary?
-
How many clock cycles are required to execute these three lines of code with forwarding?
## Rearranging Instructions
- Another way to avoid data hazards is by rearranging instructions
- Consider the following MIPS code:
```mips
lw $t1, 0($t0)
lw $t2, 4($t0)
add $t3, $t1, $t2
sw $t3, 12($t0)
lw $t4, 8($t0)
add $t5, $t1, $t4
sw $t5, 16($t0)
```
-
We need to stall the first add (because it needs `$t2`)
-
We need to stall the second add (because it needs `$t4`)
## Rearranging Instructions
- These two stalls could be avoided by rearranging the code in the following way:
```mips
lw $t1, 0($t0)
lw $t2, 4($t0)
lw $t4, 8($t0)
add $t3, $t1, $t2
sw $t3, 12($t0)
add $t5, $t1, $t4
sw $t5, 16($t0)
```
# Control Hazards
## Control Hazards
- A **control hazard** (aka branching hazard) is when the next instruction to be executed is not yet known
-
Caused by **branching** instructions such as `beq`
-
During a `beq` instruction, at what pipeline stage do we know which branch will be taken?
+
After Stage 3: EX
## Control Hazards
- One way to avoid control hazards is by stalling
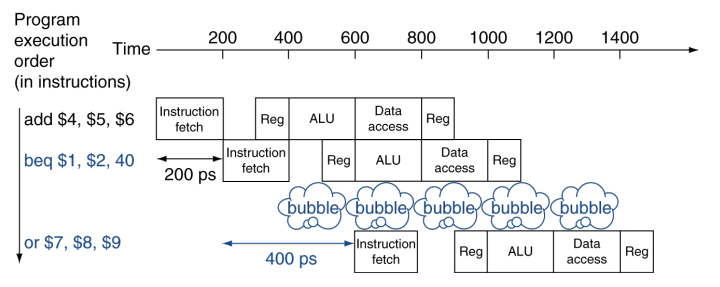
-
After every branch statement, we stall for one cycle
## Control Hazards
- The pros of the "always stall" approach are:
1.
Simple and easy to understand
2.
Will always work
-
The con of "always stall" is:
+ It is slow
## Control Hazards
- An alternative to the always stall approach is **branch prediction**
+ Make an educated guess on what the next instruction will be and execute that
+
If incorrectly guessed, "undo" the steps and go to the correct branch
## Control Hazards
- **Static branch prediction** will always predict a certain branch depending on the branching behavior
+
Predict forward branches not taken
+
Predict backward branches taken (loops)
-
**Dynamic branch prediction** keeps track of how many times a branch is taken and updates its predictions based on history
# Pipelined CPU Design
## Pipelined Control Complete