# Registers, Instructions, and System Calls --- CS 130 // 2024-09-03 # Review ## Recap of last week - MIPS: RISC architecture + 32, 32-bit registers - We wrote some a simple MIPS program in Mars + watched data change in registers and memory - Data and instructions get turned into binary and placed in memory - Covered `add`, `lw`, `sw` ```mips .data a: 9 b: 3 result: 0 .text lw $t0, a lw $t1, b add $t2, $t0, $t1 sw $t2, result ``` # More on Registers and Instructions ## MIPS Register Conventions
Name
Reg #
Usage
$zero
0
Constant 0
$v0,...
2-3
Values
$a0,...
4-7
Arguments
$t0,...
8-15
Temporaries
$s0,...
16-23
Saved (variables)
$t8,...
24-25
More temporaries
$gp
28
global pointer
$sp
29
stack pointer
$fp
30
frame pointer
$ra
31
return address
Register 1 is reserved for the assembler
Registers 26-27 are reserved for the OS
Only 8 $s registers (0..7)
Only 10 $t registers (0..9)
## Exercise - Write a MIPS program that does the equivalent of this high-level line of code ```python pay = (salary + bonus) - (health_premium + taxes) ``` ## Immediate values - A literal value like 3 in the example below is called an **immediate value** - `li` means _load immedate_ ```mips .data x: 5 .text lw $t0, x li $t1, 3 #load 3 directly into $t1 add $t0, $t1, $t0 ``` ## I-type instructions - There's also a variation of `add` where the second operand is replaced with an immediate value - `addi` means *add immediate* - it's an **I-type instruction** ```mips .data x: 5 .text lw $t0, x addi $t0, $t0, 3 ``` ## R-Type instructions - The add instruction that uses only registers is an **R-type instruction** ```mips add $t0, $t1, $t0 ``` ## System Calls - We can make **system calls** to have the system perform things like input and output - Put the system call code in `$v0` - Put argument in `$a0` (and maybe `$a1` if needed) #### Output Exercise - Run this in MARS and discuss what happens with your neighbors - What do you think `.asciiz` does? ```mips .data message: .asciiz "Hello!" .text li $v0, 4 #4 is the code for printing a string la $a0, message #the argument for the syscall syscall ``` #### Input Exercise - Run this in MARS and discuss what happens with your neighbors - Which register does the user's input go into? ```mips .data prompt: .asciiz "Enter an integer:" .text li $v0, 4 #4 is the code for printing a string la $a0, prompt #the argument for the syscall syscall li $v0, 5 #5 is the code for reading an integer syscall ``` #### Assignment: Interactive Program You now know enough to do the first assignment - [Assignment 1](../../assignments/assignment-1/): Write a program that interacts with the user and performs some kind of computation based on their input - Find other syscall codes on page B-44 of the textbook # Binary Numbers ## Let's talk about how counting works 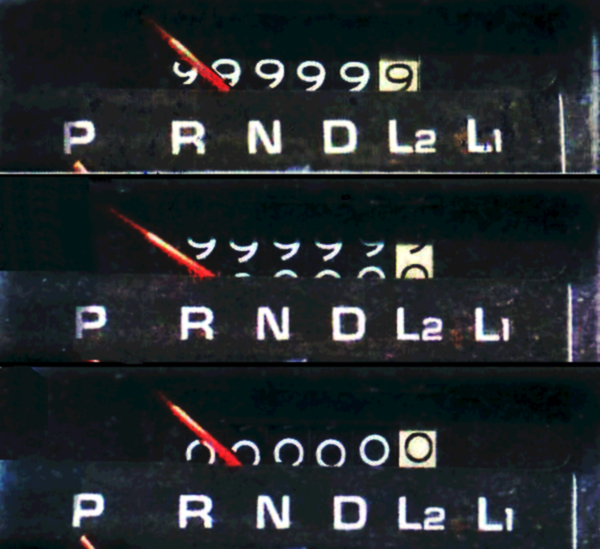 How do you count if you only have two digits?