## Introduction to # MIPS --- CS 130 // 2024-08-29 # Course Themes ## Overarching Theme - Learning how a high-level program is actually executed on your computer's processor 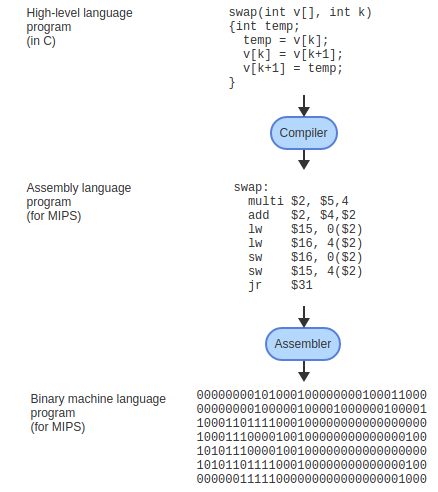 ## Trajectory of the Course 1. **Assembly language programming** 2. Digital logic 3. Processor architecture 4. The C Programming Language # Assembly Languages ## Assembly Languages - Every CPU architecture implements an **instruction set** which are the operations it natively supports - The most common CPU architectures are: + x86 (Intel/AMD) + ARM (Mobile phones, tablets, Apple's M1 chip, ...) - Instructions are extremely simple like ``` add a, b, c ``` ## Revisiting the Exercise from last time A complicated line of high-level code like this ```python pay = (salary + bonus) - (health_premium + taxes) ``` Gets translated by the *compiler* into assembly code like ```bash add basepay, salary, bonus add deductions, health_premium, taxes sub pay, basepay, deductions ``` ## RISC vs. CISC - CPU architectures are usually categorized as either "RISC" or "CISC" - RISC (Reduced Instruction Set Computing) + Simplified instructions which "do less" + ...but each instruction is highly optimized ## RISC vs. CISC - CISC (Complicated Instruction Set Computing) + Larger instruction set each of which "does more" + Optimized so that a program can be implemented with few instructions---even though those instructions may take longer to execute # MIPS ## MIPS Architecture - In this course, we will be learning the MIPS instruction set + "Microprocessor without Interlocked Pipelined Stages" - MIPS is a RISC processor with a minimalistic number of instructions - Is very similar to ARM ## Discussion Question
- what exactly are `salary` or `taxes` in this example?
```python pay = (salary + bonus) - (health_premium + taxes) ``` ## Pointers - In assembly programs, labels like `salary` or `taxes` are *pointers* - **pointer**: a stand-in for a _memory address_ - Even this is too complicated for RISC ```bash add basepay, salary, bonus ``` - You might first have to grab the data at the address indicated by the pointer _before_ you can do any operations on it. ## Grabbing data from memory - When we grab data from memory, where do we put it? - **Registers**: a holding place for data right inside the CPU - data has to be in a register before you can perform operations 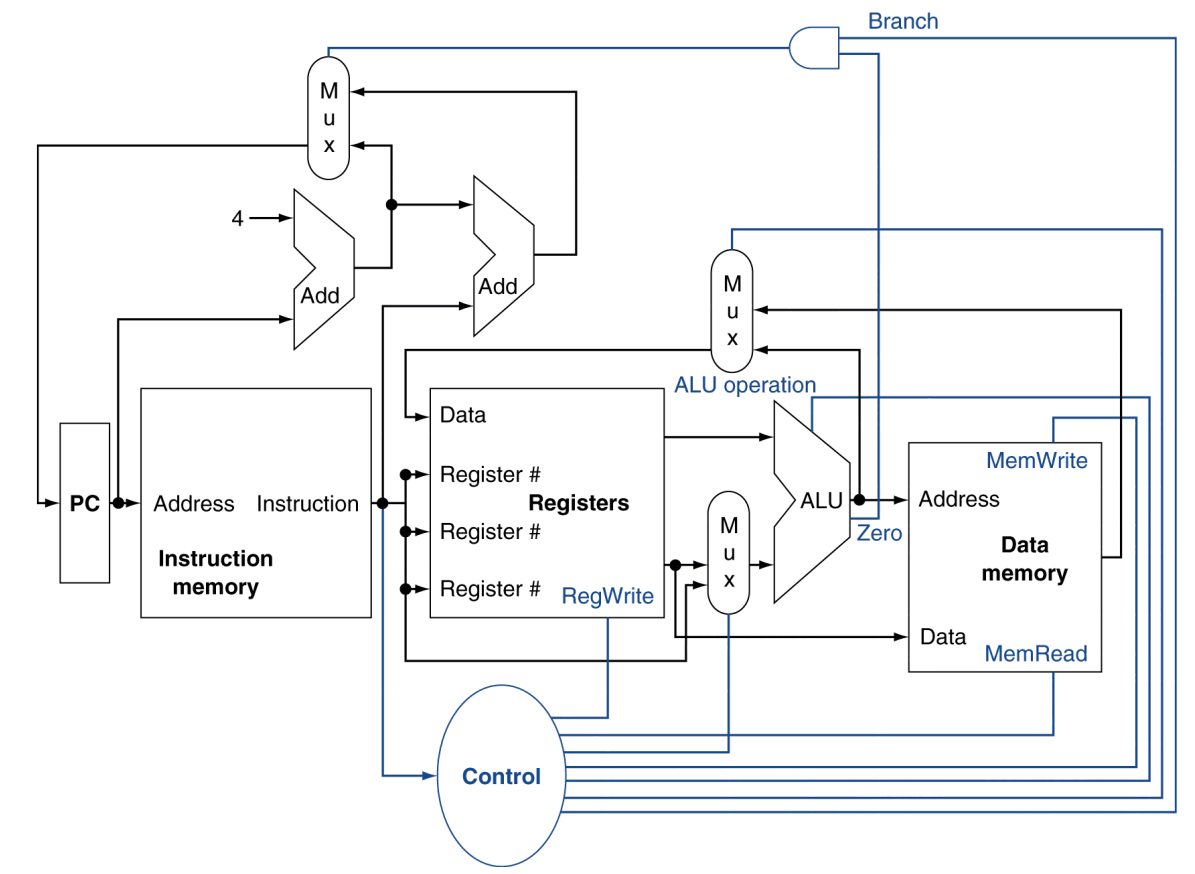 ## MIPS Architecture - Has 32 registers, each of which are 32-bits - Why not include more registers? + Cost; registers are more expensive than RAM + Performance; more registers means slower clock + Instruction size; more registers means each instruction needs more bits to identify registers #### A MIPS program in MARS 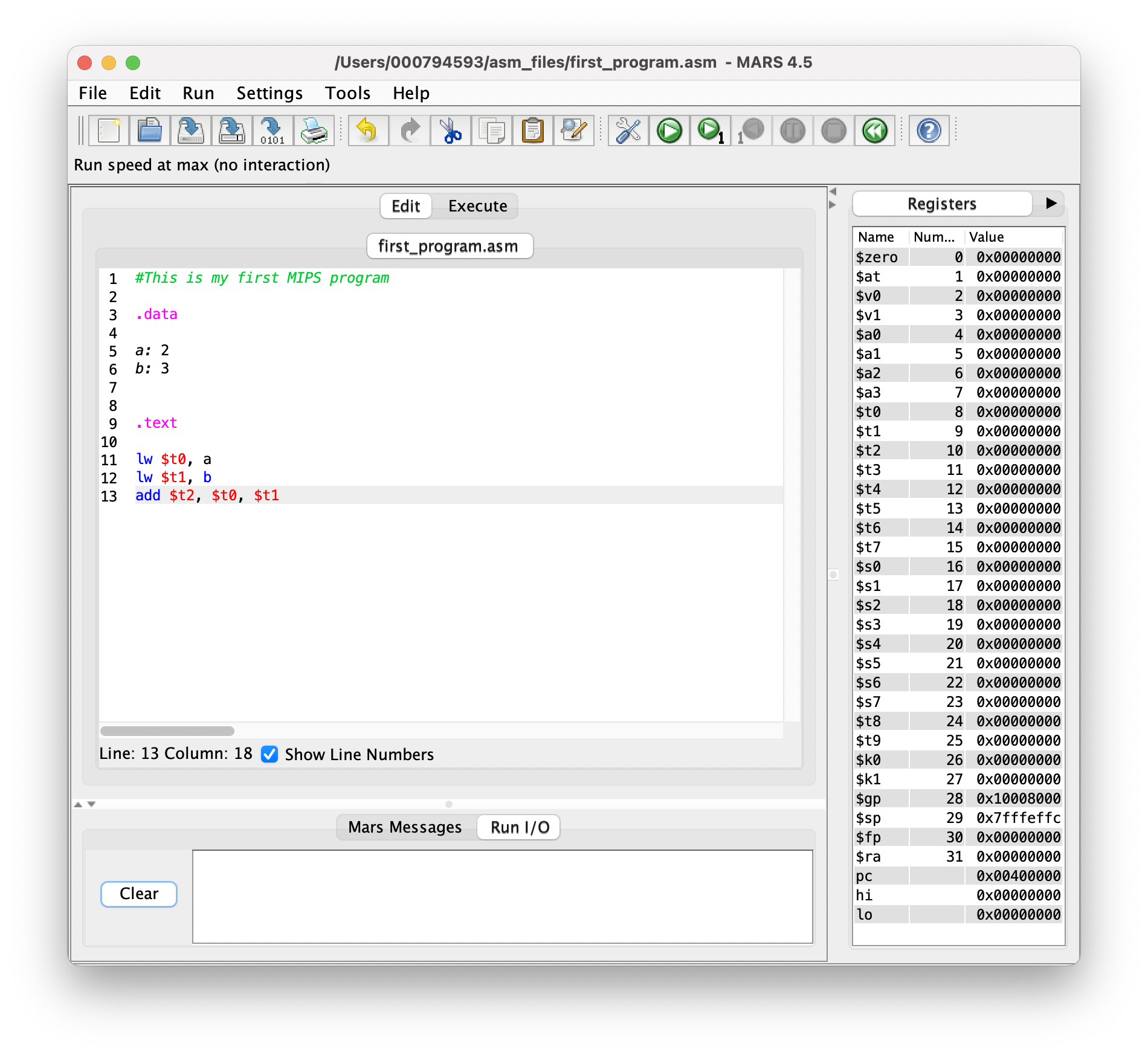 #### Things to notice in MARS - Registers have names like `$t0`, `$s2`, etc. - You can refer to a register by its name or number. - `$t0` is also `$8` - MARS can be wonky - MARS didn't play nice with OneDrive for me - you may need to create a folder for your `.asm` files in your home directory - `lw` means _load word_ - a **word** is a 32 bit value ```mips #loads a value from memory location a into register $t0 lw $t0, a ``` #### Exploration Exercise 1. Write the above program in MARS 2. What values are stored in each of the registers initially? 3. Find and press the **Assemble** button 4. What memory addresses did your *program* get stored in? 5. What memory addresses did your *data* get stored in? 6. Find and press the **Run** button 7. What values ended up in `$t0`, `$t1`, and `$t2`? Is that what you expected? 8. Change `a`'s initial value to 9 and rerun. What is in `$t2` now? What do you think is going on here? 9. Add the following to your data section ```mips result: 0 ``` 10. Add the following to the end of your text section ```mips sw $t2, result ``` 11. Rerun. Look in memory - is there a new value there? 12. What do you think `sw` means? 13. Try the **Run one step at a time** button and step through the program slowly. Watch the values change in the registers. ## MIPS Register Conventions
Name
Reg #
Usage
$zero
0
Constant 0
$v0,...
2-3
Values
$a0,...
4-7
Arguments
$t0,...
8-15
Temporaries
$s0,...
16-23
Saved (variables)
$t8,...
24-25
More temporaries
$gp
28
global pointer
$sp
29
stack pointer
$fp
30
frame pointer
$ra
31
return address
Register 1 is reserved for the assembler
Registers 26-27 are reserved for the OS
Only 8 $s registers (0..7)
Only 10 $t registers (0..9)
## Exercise - Write a MIPS program that does the equivalent of this high-level line of code ```python pay = (salary + bonus) - (health_premium + taxes) ``` ## Immediate values - A literal value like 3 in the example below is called an **immediate value** - `li` means _load immedate_ ```mips .data x: 5 .text lw $t0, x li $t1, 3 #load 3 directly into $t1 add $t0, $t1, $t0 ``` ## I-type instructions - There's also a variation of `add` where the second operand is replaced with an immediate value - `addi` means *add immediate* - it's an **I-type instruction** ```mips .data x: 5 .text lw $t0, x addi $t0, $t0, 3 ``` ## R-Type instructions - The add instruction that uses only registers is an **R-type instruction** ```mips add $t0, $t1, $t0 ``` ## System Calls - We can make **system calls** to have the system perform things like input and output - Put the system call code in `$v0` - Put argument in `$a0` (and maybe `$a1` if needed) #### Output Exercise - Run this in MARS and discuss what happens with your neighbors - What do you think `.asciiz` does? ```mips .data message: .asciiz "Hello!" .text li $v0, 4 #4 is the code for printing a string la $a0, message #the argument for the syscall syscall ``` #### Input Exercise - Run this in MARS and discuss what happens with your neighbors - Which register does the user's input go into? ```mips .data prompt: .asciiz "Enter an integer:" .text li $v0, 4 #4 is the code for printing a string la $a0, prompt #the argument for the syscall syscall li $v0, 5 #5 is the code for reading an integer syscall ``` #### Interactive Program Exercise - [Assignment 1](../../assignments/assignment-1/): Write a program that interacts with the user and performs some kind of computation based on their input - Find other syscall codes on page B-44 of the textbook